Oracle SQL: Enhancing Your Queries With IF AND Statements
What is Oracle SQL IF and how can it improve your data management?
Oracle SQL IF is a powerful statement used to control the flow of execution in Oracle SQL code. It allows you to conditionally execute blocks of code based on the evaluation of a specified condition. The syntax of the Oracle SQL IF statement is as follows:
IF condition THEN -- code to be executed if condition is TRUEELSE -- code to be executed if condition is FALSEEND IF;
The Oracle SQL IF statement can be used to implement a variety of conditional logic, such as:
- Checking for the existence of a value
- Comparing values
- Determining the data type of a value
The Oracle SQL IF statement is a versatile and powerful tool that can be used to improve the efficiency and accuracy of your Oracle SQL code. By using the Oracle SQL IF statement, you can conditionally execute blocks of code based on the evaluation of a specified condition. This allows you to create more dynamic and responsive Oracle SQL code that can handle a variety of different scenarios.
In addition to the basic Oracle SQL IF statement, there are also several other variations of the Oracle SQL IF statement that can be used to implement more complex conditional logic. These variations include the Oracle SQL IF-ELSE statement, the Oracle SQL IF-ELSEIF statement, and the Oracle SQL CASE statement.
Oracle SQL IF and
The Oracle SQL IF statement is a powerful tool that allows you to control the flow of execution in your Oracle SQL code. It allows you to conditionally execute blocks of code based on the evaluation of a specified condition. The Oracle SQL IF statement can be used to implement a variety of conditional logic, such as:
- Checking for the existence of a value
- Comparing values
- Determining the data type of a value
- Executing different code paths based on the value of a variable
- Handling errors and exceptions
- Creating more dynamic and responsive Oracle SQL code
The Oracle SQL IF statement is a versatile and powerful tool that can be used to improve the efficiency and accuracy of your Oracle SQL code. By using the Oracle SQL IF statement, you can conditionally execute blocks of code based on the evaluation of a specified condition. This allows you to create more dynamic and responsive Oracle SQL code that can handle a variety of different scenarios.
In addition to the basic Oracle SQL IF statement, there are also several other variations of the Oracle SQL IF statement that can be used to implement more complex conditional logic. These variations include the Oracle SQL IF-ELSE statement, the Oracle SQL IF-ELSEIF statement, and the Oracle SQL CASE statement.Checking for the existence of a value
One of the most common uses of the Oracle SQL IF statement is to check for the existence of a value. This can be useful in a variety of situations, such as:
- Determining whether a record exists in a table
For example, the following Oracle SQL statement checks for the existence of a record in thecustomers
table where thecustomer_id
is equal to 1:IF EXISTS (SELECT * FROM customers WHERE customer_id = 1) THEN -- code to be executed if the record existsELSE -- code to be executed if the record does not existEND IF;
- Checking whether a column value is NULL
For example, the following Oracle SQL statement checks whether theorder_date
column in theorders
table is NULL:IF order_date IS NULL THEN -- code to be executed if the order_date column is NULLELSE -- code to be executed if the order_date column is not NULLEND IF;
- Checking whether a variable has been assigned a value
For example, the following Oracle SQL statement checks whether the@customer_id
variable has been assigned a value:IF @customer_id IS NOT NULL THEN -- code to be executed if the @customer_id variable has been assigned a valueELSE -- code to be executed if the @customer_id variable has not been assigned a valueEND IF;
- Checking whether a function returns a value
For example, the following Oracle SQL statement checks whether theGET_CUSTOMER_NAME()
function returns a value:IF GET_CUSTOMER_NAME() IS NOT NULL THEN -- code to be executed if the GET_CUSTOMER_NAME() function returns a valueELSE -- code to be executed if the GET_CUSTOMER_NAME() function does not return a valueEND IF;
By using the Oracle SQL IF statement to check for the existence of a value, you can create more dynamic and responsive Oracle SQL code that can handle a variety of different scenarios.
Comparing values
Another common use of the Oracle SQL IF statement is to compare values. This can be useful in a variety of situations, such as:
- Determining whether two values are equal
For example, the following Oracle SQL statement checks whether thecustomer_id
column in thecustomers
table is equal to 1:IF customer_id = 1 THEN -- code to be executed if the customer_id column is equal to 1ELSE -- code to be executed if the customer_id column is not equal to 1END IF;
- Comparing two columns in a table
For example, the following Oracle SQL statement checks whether theorder_date
column in theorders
table is greater than theship_date
column:IF order_date > ship_date THEN -- code to be executed if the order_date column is greater than the ship_date columnELSE -- code to be executed if the order_date column is not greater than the ship_date columnEND IF;
- Comparing a variable to a value
For example, the following Oracle SQL statement checks whether the@customer_id
variable is equal to 1:IF @customer_id = 1 THEN -- code to be executed if the @customer_id variable is equal to 1ELSE -- code to be executed if the @customer_id variable is not equal to 1END IF;
- Comparing the result of a function to a value
For example, the following Oracle SQL statement checks whether theGET_CUSTOMER_NAME()
function returns a value that is equal to 'John Doe':IF GET_CUSTOMER_NAME() = 'John Doe' THEN -- code to be executed if the GET_CUSTOMER_NAME() function returns the value 'John Doe'ELSE -- code to be executed if the GET_CUSTOMER_NAME() function does not return the value 'John Doe'END IF;
By using the Oracle SQL IF statement to compare values, you can create more dynamic and responsive Oracle SQL code that can handle a variety of different scenarios.
Using Oracle SQL IF to Determine the Data Type of a Value
Oracle SQL IF statements can be used in conjunction with data type functions to determine the data type of a value. This may be beneficial in scenarios where you want to process values differently based on their data type. For instance, suppose you have a column that can contain values of type integer, string, or date. You can use an IF statement to identify the data type of each value and perform the appropriate processing.
Here's an example using the `TO_CHAR()` function to convert a value to a string and the `LENGTH()` function to determine the length of the resulting string. This can be useful for identifying the data type of a value that may not be immediately apparent.
IF LENGTH(TO_CHAR()) = 10 THEN -- The value is a date.ELSEIF LENGTH(TO_CHAR()) > 10 THEN -- The value is a string.ELSE -- The value is an integer.END IF;
By understanding the data type of a value, you can create more robust and efficient Oracle SQL code that can handle a variety of different data types.
Executing different code paths based on the value of a variable
In Oracle SQL, the IF statement allows you to execute different code paths based on the value of a variable. This is a powerful capability that can be used to create more dynamic and responsive code.
One common use case for the IF statement is to check the value of a variable to determine which SQL statement to execute. For example, the following code checks the value of the `@status` variable to determine whether to insert or update a record in the `customers` table:
IF @status = 'new' THEN -- Insert a new record into the customers table ELSE -- Update the existing record in the customers table END IF;
The IF statement can also be used to control the flow of execution within a PL/SQL block. For example, the following code uses an IF statement to conditionally execute a loop:
DECLARE -- Declare a variable to store the loop counter i NUMBER := 0; BEGIN -- Loop through the rows in the customers table FOR customer IN (SELECT * FROM customers) LOOP -- Increment the loop counter i := i + 1; -- Check if the loop counter is greater than 10 IF i > 10 THEN -- Exit the loop EXIT; END IF; -- Process the current customer -- ... END LOOP; END;
The IF statement is a versatile tool that can be used to control the flow of execution in Oracle SQL code. By understanding how to use the IF statement, you can create more dynamic and responsive code that can handle a variety of different scenarios.
Handling errors and exceptions
In Oracle SQL, the IF statement can be used to handle errors and exceptions. This is a critical capability that can help to ensure the reliability and robustness of your code.
One common use case for the IF statement is to check for errors that occur during the execution of a SQL statement. For example, the following code checks for errors that occur during the execution of the `INSERT` statement:
BEGIN -- Insert a new record into the customers table INSERT INTO customers (customer_id, customer_name) VALUES (10, 'John Doe'); -- Check for errors IF SQL%ROWCOUNT = 0 THEN -- An error occurred during the INSERT statement -- ... END IF;END;
The IF statement can also be used to handle exceptions that occur during the execution of a PL/SQL block. For example, the following code uses an IF statement to handle the `NO_DATA_FOUND` exception:
BEGIN -- Declare a cursor to select rows from the customers table DECLARE cursor c_customers IS SELECT * FROM customers; -- Open the cursor OPEN c_customers; -- Loop through the rows in the cursor LOOP FETCH c_customers INTO customer_id, customer_name; -- Check if the cursor has reached the end of the results IF c_customers%NOTFOUND THEN -- The cursor has reached the end of the results EXIT; END IF; -- Process the current customer -- ... END LOOP; -- Close the cursor CLOSE c_customers;END;
The IF statement is a versatile tool that can be used to handle errors and exceptions in Oracle SQL code. By understanding how to use the IF statement, you can create more robust and reliable code that can handle a variety of different scenarios.
Creating more dynamic and responsive Oracle SQL code
In the realm of database management, crafting dynamic and responsive Oracle SQL code is paramount for ensuring efficient and adaptable data processing. The Oracle SQL IF statement plays a pivotal role in achieving this objective by providing conditional execution capabilities.
- Conditional Execution
The IF statement allows developers to conditionally execute blocks of code based on the evaluation of a specified condition. This enables the creation of code that can adapt to different scenarios and handle varying data conditions. - Error Handling
The IF statement can be used to handle errors and exceptions that may arise during the execution of SQL statements. By checking for specific error codes or conditions, developers can gracefully handle errors and provide appropriate responses. - Dynamic Query Construction
The IF statement can be employed to construct dynamic SQL queries based on user input or runtime conditions. This allows for the creation of queries that can be tailored to specific requirements, improving the flexibility and efficiency of data retrieval. - Procedural Control
Within PL/SQL blocks, the IF statement provides procedural control, enabling developers to create complex control structures and implement branching logic. This enhances the overall structure and readability of the code.
By harnessing the power of the Oracle SQL IF statement, developers can create code that is not only efficient but also adaptable to changing requirements. The ability to conditionally execute code, handle errors, construct dynamic queries, and implement procedural control empowers developers to build robust and responsive Oracle SQL applications.
FAQs on Oracle SQL IF and
The Oracle SQL IF statement is a powerful tool that allows developers to control the flow of execution in Oracle SQL code. It enables conditional execution of code blocks based on the evaluation of a specified condition. Here are some frequently asked questions about the Oracle SQL IF statement:
Question 1: What are the different types of Oracle SQL IF statements?
There are three main types of Oracle SQL IF statements:
- IF-THEN statement: Executes a block of code if the specified condition is TRUE.
- IF-THEN-ELSE statement: Executes a block of code if the specified condition is TRUE, and a different block of code if the condition is FALSE.
- IF-THEN-ELSEIF statement: Executes a block of code if the specified condition is TRUE, and a different block of code if the condition is FALSE. The ELSEIF clause can be used to specify multiple conditions.
Question 2: How can I use the Oracle SQL IF statement to handle errors?
The Oracle SQL IF statement can be used to handle errors by checking for specific error codes or conditions. If an error occurs, the IF statement can execute a block of code to handle the error and provide an appropriate response.
Question 3: Can I use the Oracle SQL IF statement to construct dynamic queries?
Yes, the Oracle SQL IF statement can be used to construct dynamic queries based on user input or runtime conditions. This allows for the creation of queries that can be tailored to specific requirements, improving the flexibility and efficiency of data retrieval.
Question 4: How does the Oracle SQL IF statement differ from the CASE statement?
The Oracle SQL IF statement is used for conditional execution of code blocks, while the CASE statement is used for evaluating multiple conditions and returning a specific value based on the matching condition.
Question 5: What are some best practices for using the Oracle SQL IF statement?
Some best practices for using the Oracle SQL IF statement include:
- Use clear and concise conditions.
- Use proper indentation to improve readability.
- Handle all possible cases, including the ELSE case.
- Use the IF statement judiciously to avoid overly complex code.
Question 6: Where can I find more information about the Oracle SQL IF statement?
You can find more information about the Oracle SQL IF statement in the Oracle SQL documentation or by searching for tutorials and examples online.
Conclusion on Oracle SQL IF and
The Oracle SQL IF statement is a powerful and versatile tool that allows developers to control the flow of execution in Oracle SQL code. It enables conditional execution of code blocks based on the evaluation of a specified condition. The IF statement can be used to implement a wide range of conditional logic, including checking for the existence of a value, comparing values, and handling errors.
By understanding how to use the Oracle SQL IF statement, developers can create more dynamic and responsive Oracle SQL code that can handle a variety of different scenarios. The IF statement is an essential tool for any Oracle SQL developer, and it is important to understand how to use it effectively.
Why Do Cats Like Each Other's Butts? The Secret Language Of Cats
Where To Find Signature Select Brand Products
Ultimate Guide To Change Language Settings In Windows 11

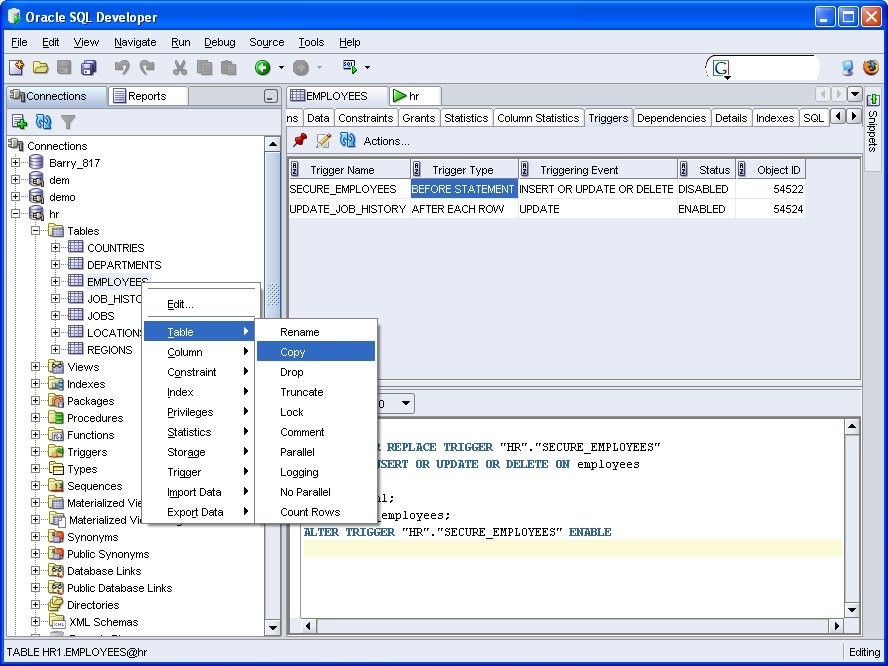