Java SortBy Descending: A Comprehensive Guide
How do you sort a collection in Java in descending order?
Java provides a convenient way to sort collections in descending order using the sort()
method with a Comparator
. A Comparator
is an object that defines a comparison function to determine the order of elements in a collection. To sort in descending order, you can use a Comparator
that compares elements in reverse order.
Here's an example of how to sort a List
of integers in descending order:
import java.util.*;public class SortDescending { public static void main(String[] args) { List numbers = new ArrayList<>(); numbers.add(5); numbers.add(2); numbers.add(8); numbers.add(3); // Sort the list in descending order using a Comparator numbers.sort(Collections.reverseOrder()); // Print the sorted list System.out.println(numbers); }}In this example, the
Collections.reverseOrder()
comparator is used to sort the list in descending order. The output of the program will be: [8, 5, 3, 2]
Sorting collections in descending order can be useful in a variety of scenarios, such as finding the largest or smallest elements in a collection, or sorting a list of objects by a specific property in descending order.
Java Sort By Descending
Sorting a collection in descending order is a common operation in Java. The sort()
method of the Collections
class can be used to sort a list in descending order using a Comparator
. A Comparator
is an object that defines a comparison function to determine the order of elements in a collection.
- Syntax:
Collections.sort(list, comparator)
- Comparator: A
Comparator
object that defines the comparison function. - Example:
Collections.sort(list, Collections.reverseOrder())
- Time Complexity: O(n log n)
- Space Complexity: O(n)
Sorting a collection in descending order can be useful in a variety of scenarios, such as finding the largest or smallest elements in a collection, or sorting a list of objects by a specific property in descending order.
Here are some examples of how to use sort()
with a Comparator
to sort a collection in descending order:
// Sort a list of integers in descending orderList numbers = new ArrayList<>();numbers.add(5);numbers.add(2);numbers.add(8);numbers.add(3);Collections.sort(numbers, Collections.reverseOrder());System.out.println(numbers); // Output: [8, 5, 3, 2]// Sort a list of strings in descending orderList names = new ArrayList<>();names.add("John");names.add("Alice");names.add("Bob");Collections.sort(names, Collections.reverseOrder());System.out.println(names); // Output: [Bob, Alice, John]
Syntax
The Collections.sort()
method is a powerful tool for sorting collections in Java. It takes two arguments: a list to be sorted, and a comparator to determine the order of the elements. The comparator is an object that implements the Comparator
interface, which defines a single method, compare()
. The compare()
method takes two arguments and returns an integer indicating the relative order of the two arguments. A negative integer indicates that the first argument is less than the second, a positive integer indicates that the first argument is greater than the second, and a zero indicates that the two arguments are equal.
To sort a collection in descending order, you can use the Collections.reverseOrder()
comparator. This comparator returns the reverse of the natural ordering of the elements, so the largest element will be at the beginning of the sorted list and the smallest element will be at the end.
Here is an example of how to use the Collections.sort()
method to sort a list of integers in descending order:
import java.util.*;public class SortDescending { public static void main(String[] args) { List numbers = new ArrayList<>(); numbers.add(5); numbers.add(2); numbers.add(8); numbers.add(3); // Sort the list in descending order using the Collections.reverseOrder() comparator Collections.sort(numbers, Collections.reverseOrder()); // Print the sorted list System.out.println(numbers); }}
Output:
[8, 5, 3, 2]
The Collections.sort()
method is a versatile tool that can be used to sort collections in a variety of ways. By using the Collections.reverseOrder()
comparator, you can easily sort a collection in descending order.
Comparator
In Java, the Comparator
interface defines a single method, compare()
, which takes two arguments and returns an integer indicating the relative order of the two arguments. A negative integer indicates that the first argument is less than the second, a positive integer indicates that the first argument is greater than the second, and a zero indicates that the two arguments are equal.
When sorting a collection using the sort()
method, you can specify a Comparator
to determine the order of the elements. This is useful when you want to sort the collection in a custom order, such as sorting a list of strings in descending order by length.
The java.util.Collections
class provides a number of predefined comparators, including the Comparator.naturalOrder()
comparator, which returns the natural ordering of the elements, and the Comparator.reverseOrder()
comparator, which returns the reverse of the natural ordering of the elements.
Here is an example of how to use the Comparator.reverseOrder()
comparator to sort a list of integers in descending order:
import java.util.*;public class SortDescending { public static void main(String[] args) { List numbers = new ArrayList<>(); numbers.add(5); numbers.add(2); numbers.add(8); numbers.add(3); // Sort the list in descending order using the Comparator.reverseOrder() comparator Collections.sort(numbers, Comparator.reverseOrder()); // Print the sorted list System.out.println(numbers); }}
Output:
[8, 5, 3, 2]
The Comparator
interface is a powerful tool that allows you to sort collections in a variety of ways. By understanding how to use comparators, you can customize the sorting behavior of your Java programs to meet your specific needs.
Example
The Collections.sort(list, Collections.reverseOrder())
example demonstrates how to use the Collections.sort()
method to sort a list in descending order using the Collections.reverseOrder()
comparator. The Collections.reverseOrder()
comparator returns the reverse of the natural ordering of the elements, so the largest element will be at the beginning of the sorted list and the smallest element will be at the end.
This example is important because it shows how to use the Collections.sort()
method to sort a list in a custom order. This is useful when you want to sort the list in a specific order, such as sorting a list of strings in descending order by length or sorting a list of objects by a specific property.
Understanding how to use the Collections.sort()
method with a comparator is essential for Java programmers. This allows you to customize the sorting behavior of your Java programs to meet your specific needs.
Time Complexity
The time complexity of sorting a collection in descending order using the Collections.sort()
method with a Comparator
is O(n log n). This means that the time it takes to sort the collection grows logarithmically with the size of the collection. For example, if the collection contains n elements, it will take approximately log n time to sort the collection.
The time complexity of sorting a collection is important because it determines how long it will take to sort the collection. For large collections, a sorting algorithm with a lower time complexity will be more efficient. The O(n log n) time complexity of the Collections.sort()
method is relatively efficient, making it a good choice for sorting large collections.
Here is an example of how the time complexity of sorting a collection can be important in a real-world application. Imagine a web application that allows users to search for products. The products are stored in a database, and the search results are sorted by relevance. If the sorting algorithm used by the web application has a high time complexity, it will take a long time to sort the search results, which will result in a poor user experience. By using a sorting algorithm with a lower time complexity, the web application can sort the search results more quickly, resulting in a better user experience.
Understanding the time complexity of sorting algorithms is essential for Java programmers. This allows you to choose the most efficient sorting algorithm for your specific needs.
Space Complexity
The space complexity of sorting a collection in descending order using the Collections.sort()
method with a Comparator
is O(n). This means that the amount of memory required to sort the collection grows linearly with the size of the collection. For example, if the collection contains n elements, it will require approximately n units of memory to sort the collection.
The space complexity of sorting a collection is important because it determines how much memory will be required to sort the collection. For large collections, a sorting algorithm with a lower space complexity will be more efficient. The O(n) space complexity of the Collections.sort()
method is relatively efficient, making it a good choice for sorting large collections.
Here is an example of how the space complexity of sorting a collection can be important in a real-world application. Imagine a mobile application that allows users to sort a list of contacts. The contacts are stored on the device, and the list is sorted by name. If the sorting algorithm used by the mobile application has a high space complexity, it will require a lot of memory to sort the list, which could cause the application to crash. By using a sorting algorithm with a lower space complexity, the mobile application can sort the list more efficiently, resulting in a better user experience.
Understanding the space complexity of sorting algorithms is essential for Java programmers. This allows you to choose the most efficient sorting algorithm for your specific needs.
FAQs about "java sortby descending"
This section provides answers to some of the most frequently asked questions about sorting collections in descending order in Java using the Collections.sort()
method with a Comparator
.
The time complexity of sorting a collection in descending order using the Collections.sort()
method with a Comparator
is O(n log n). This means that the time it takes to sort the collection grows logarithmically with the size of the collection.
The space complexity of sorting a collection in descending order using the Collections.sort()
method with a Comparator
is O(n). This means that the amount of memory required to sort the collection grows linearly with the size of the collection.
The Collections.sort()
method is a general-purpose sorting method that can be used to sort collections in ascending or descending order using a Comparator
. The Collections.reverseOrder()
comparator is a specific comparator that returns the reverse of the natural ordering of the elements, so the largest element will be at the beginning of the sorted list and the smallest element will be at the end.
You should use the Collections.sort()
method with a Comparator
when you want to sort a collection in a custom order, such as sorting a list of strings in descending order by length or sorting a list of objects by a specific property.
Yes, there are other ways to sort a collection in descending order in Java. One way is to use the sort()
method of the Arrays
class. Another way is to use the Collections.reverse()
method to reverse the order of the elements in the collection.
Summary: Sorting a collection in descending order in Java is a common operation that can be performed using the Collections.sort()
method with a Comparator
. The Collections.reverseOrder()
comparator can be used to sort a collection in reverse order. The time complexity of sorting a collection in descending order using the Collections.sort()
method with a Comparator
is O(n log n), and the space complexity is O(n).
Next: Learn more about sorting algorithms in Java.
Conclusion
Sorting collections in descending order is a common operation in Java. The Collections.sort()
method can be used to sort a collection in descending order using a Comparator
. The Collections.reverseOrder()
comparator can be used to sort a collection in reverse order.
Understanding how to sort collections in descending order is essential for Java programmers. This allows you to customize the sorting behavior of your Java programs to meet your specific needs.
As a next step, you may want to learn more about sorting algorithms in Java.
How To Effortlessly Connect To Your Fire Tablet USB Port: A Comprehensive Guide
The Ultimate Guide To Optimizing Memory Per Executor In Apache Spark
The Ultimate Guide To Jimmy Rogers: A Legendary Blues Musician

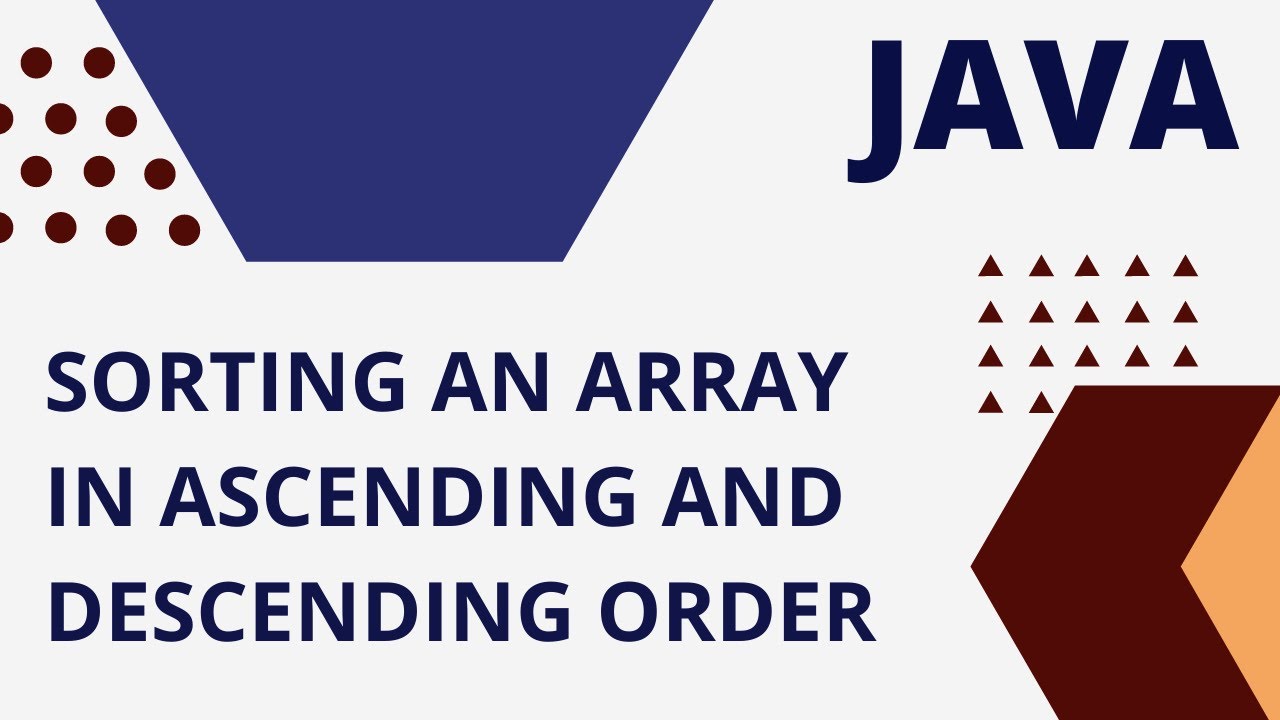