Secure Authentication With C#: A Comprehensive Guide For Developers
What is authentication, and why is it essential?
Authentication is the process of verifying the identity of a user or system. It is a critical security measure that helps to protect against unauthorized access to data and resources. Authentication can be performed using a variety of methods, including passwords, biometrics, and digital certificates.
Authentication is essential for both online and offline systems. In an online environment, authentication helps to protect against unauthorized access to websites, applications, and data. In an offline environment, authentication helps to protect against unauthorized access to physical resources, such as buildings and computer systems.
There are many different types of authentication methods available. The most common type of authentication method is password-based authentication. Password-based authentication requires users to enter a password in order to gain access to a system. Other types of authentication methods include biometric authentication, which uses unique physical characteristics to identify users, and digital certificate authentication, which uses digital certificates to verify the identity of users.
The choice of authentication method depends on the level of security required. For systems that require a high level of security, such as financial systems, two-factor authentication is often used. Two-factor authentication requires users to provide two different pieces of evidence in order to gain access to a system, such as a password and a security token.
Authentication in C#
Authentication is the process of verifying the identity of a user or system. It is a critical security measure that helps to protect against unauthorized access to data and resources.
- Methods: C# provides several authentication methods, including password-based authentication, biometric authentication, and digital certificate authentication.
- Forms: Authentication can be performed in various forms, such as user authentication, service authentication, and device authentication.
- Security: Authentication plays a vital role in maintaining the security and integrity of applications and systems.
- Authorization: Authentication is often used in conjunction with authorization to control access to specific resources or operations.
- Best Practices: Implementing strong authentication practices, such as using strong passwords and multi-factor authentication, is crucial for enhancing security.
- Challenges: Authentication systems can face challenges such as phishing attacks, brute-force attacks, and social engineering.
- Trends: Emerging authentication technologies, such as biometrics and behavioral analysis, are gaining traction.
In summary, authentication in C# is essential for securing applications and systems by verifying the identity of users or systems. By understanding the different methods, forms, and best practices of authentication, developers can implement robust authentication mechanisms to protect against unauthorized access and maintain the integrity of their applications.
Methods
In the context of "authentication c#", the various authentication methods provided by C# play a pivotal role in securing applications and systems. These methods offer a range of options to suit different security requirements and use cases.
- Password-based Authentication:
This is the most common authentication method, where users are required to provide a password to gain access. C# provides support for password hashing and encryption to protect passwords from unauthorized access.
- Biometric Authentication:
Biometric authentication methods leverage unique physical characteristics, such as fingerprints, facial recognition, or voice patterns, to verify a user's identity. C# supports integration with biometric authentication devices and frameworks.
- Digital Certificate Authentication:
Digital certificates are electronic credentials that bind a user's identity to a public key. C# provides APIs for working with digital certificates, enabling secure authentication and encryption.
The choice of authentication method depends on factors such as the sensitivity of the data being protected, the level of security required, and the user experience. By leveraging the diverse authentication methods available in C#, developers can implement robust and secure authentication mechanisms tailored to their specific application needs.
Forms
In the context of "authentication c#", the different forms of authentication play a crucial role in addressing diverse authentication scenarios and requirements.
- User Authentication:
This is the most common form of authentication, where individual users are authenticated to access applications or systems. C# provides support for user authentication mechanisms such as password-based authentication, multi-factor authentication, and social media login.
- Service Authentication:
This form of authentication is used when one service needs to access another service. C# supports service authentication mechanisms such as OAuth and JWT (JSON Web Tokens) to securely exchange authorization credentials between services.
- Device Authentication:
Device authentication is used to verify the identity of a device, such as a smartphone or IoT device. C# supports device authentication mechanisms such as certificate-based authentication and hardware-based authentication.
By understanding and leveraging the different forms of authentication available in C#, developers can implement comprehensive and secure authentication mechanisms that cater to the specific requirements of their applications and systems.
Security
In the context of "authentication c#", the significance of security cannot be overstated. Authentication serves as the cornerstone of robust security measures, safeguarding applications and systems against unauthorized access and malicious activities.
When authentication is effectively implemented using C#, it ensures that only authorized users or entities can access sensitive data, resources, and functionalities. This plays a critical role in preventing data breaches, identity theft, and other security threats. By verifying the identity of users, authentication helps establish a trusted environment, where the integrity and confidentiality of information are maintained.
Real-life examples abound where robust authentication mechanisms have proven invaluable. In the realm of online banking, authentication plays a crucial role in protecting users' financial assets by ensuring that only the rightful account holders can access their funds. Similarly, in healthcare systems, authentication safeguards patient data, ensuring that only authorized medical professionals can view and modify sensitive medical records.
Understanding the connection between "Security: Authentication plays a vital role in maintaining the security and integrity of applications and systems." and "authentication c#" empowers developers to create secure and reliable applications. By leveraging the authentication features and best practices provided by C#, developers can implement robust authentication mechanisms tailored to the specific security requirements of their applications and systems.
Authorization
In the realm of "authentication c#", the interplay between authentication and authorization is of paramount importance. While authentication verifies a user or entity's identity, authorization determines their level of access to specific resources or operations within a system.
Authorization plays a vital role in enforcing access control policies, ensuring that users can only perform actions and access data that are appropriate for their roles and permissions. This is particularly critical in multi-user environments, where it is essential to prevent unauthorized individuals from gaining access to sensitive information or performing unauthorized actions.
For instance, in an e-commerce application, authentication verifies the identity of a user logging into the system. Authorization then determines whether the user has the necessary permissions to perform specific actions, such as adding items to their shopping cart, making purchases, or managing their account details.
Understanding the connection between authentication and authorization is essential for developers building secure and reliable applications. By implementing robust authentication and authorization mechanisms using C#, developers can effectively control access to resources and operations, ensuring that only authorized users can perform specific actions.
This understanding also empowers developers to create fine-grained access control systems that cater to the specific requirements of their applications and systems. By leveraging the capabilities of C# and adhering to best practices, developers can implement secure and scalable authorization mechanisms that protect sensitive data and maintain the integrity of their applications.
Best Practices
In the context of "authentication c#", implementing robust authentication practices is paramount to safeguarding applications and systems from unauthorized access and malicious activities. This section explores some key best practices that developers should adopt when designing and implementing authentication mechanisms using C#.
- Use Strong Passwords:
Enforce the use of strong passwords by implementing password complexity rules. Strong passwords should be at least 12 characters long and include a combination of uppercase and lowercase letters, numbers, and special characters. Avoid common words or phrases that can be easily guessed.
- Implement Multi-Factor Authentication (MFA):
Incorporate MFA to add an extra layer of security to the authentication process. MFA requires users to provide two or more different forms of authentication, such as a password and a one-time code sent to their mobile phone or email address. This makes it significantly harder for attackers to gain unauthorized access, even if they have obtained a user's password.
- Use Secure Storage for Sensitive Data:
When storing sensitive authentication information, such as passwords and tokens, use secure storage mechanisms provided by C# and the .NET Framework. Avoid storing authentication data in plain text or in easily accessible locations. Consider using encryption and hashing algorithms to protect sensitive data from unauthorized access.
- Implement Session Management Best Practices:
Enforce secure session management practices to prevent session hijacking and other attacks. Use strong session identifiers, set appropriate session timeouts, and implement mechanisms to invalidate sessions when necessary. Regularly review and update session management policies to address evolving threats and vulnerabilities.
By adhering to these best practices and leveraging the capabilities of C#, developers can significantly enhance the security of their applications and systems. Implementing strong authentication mechanisms is a crucial step towards protecting against unauthorized access and ensuring the integrity of data and resources.
Challenges
Authentication systems, including those built with C#, are not immune to various challenges and attacks. Understanding these challenges is crucial for implementing robust and secure authentication mechanisms.
Phishing attacks attempt to trick users into revealing their credentials by disguising malicious websites or emails as legitimate ones. Brute-force attacks involve repeatedly trying different combinations of usernames and passwords to gain unauthorized access. Social engineering attacks exploit human psychology to manipulate users into divulging sensitive information.
In the context of "authentication c#", being aware of these challenges allows developers to take proactive measures to safeguard their applications and systems. By implementing strong authentication practices, such as multi-factor authentication and secure password storage, developers can make it more difficult for attackers to succeed.
For instance, in an e-commerce application built with C#, implementing strong password hashing algorithms and enforcing complex password requirements can help protect against brute-force attacks. Additionally, incorporating MFA can add an extra layer of security, making it harder for attackers to gain unauthorized access even if they obtain a user's password.
Understanding the challenges faced by authentication systems and employing appropriate countermeasures are essential for building secure and reliable applications with C#. By staying informed about the latest threats and trends, developers can proactively address these challenges and enhance the overall security of their applications and systems.
Trends
In the realm of "authentication c#", the emergence of cutting-edge authentication technologies is revolutionizing the way we authenticate users and secure systems. Biometrics and behavioral analysis are two such technologies that have garnered significant attention and are shaping the future of authentication.
- Biometrics:
Biometric authentication leverages unique physical or behavioral characteristics of individuals, such as fingerprints, facial features, or voice patterns, to verify their identity. This technology offers enhanced security compared to traditional password-based methods, as biometric traits are difficult to replicate or steal.
- Behavioral Analysis:
Behavioral analysis examines patterns of user behavior, such as typing rhythm, mouse movements, and application usage, to authenticate users. This approach is based on the premise that individuals exhibit unique behavioral patterns that can be used to identify them. By analyzing these patterns, behavioral analysis systems can detect anomalies and flag suspicious activities, providing an additional layer of security.
The integration of biometrics and behavioral analysis into authentication mechanisms using C# empowers developers to create highly secure and user-friendly authentication systems. These technologies offer a range of benefits, including improved security, reduced reliance on passwords, and enhanced user convenience.
Frequently Asked Questions about Authentication in C#
This section addresses common questions and misconceptions regarding authentication in C# to provide a comprehensive understanding of the topic.
Question 1: What are the different types of authentication methods supported by C#?
C# supports a range of authentication methods, including password-based authentication, biometric authentication, and digital certificate authentication, catering to diverse security requirements.
Question 2: Why is multi-factor authentication (MFA) recommended for enhanced security?
MFA adds an extra layer of security by requiring users to provide multiple forms of authentication, making it significantly harder for attackers to gain unauthorized access, even if they obtain a user's password.
Question 3: How can I securely store authentication information in C# applications?
C# provides secure storage mechanisms to safeguard sensitive authentication data, such as passwords and tokens. Developers should avoid storing this information in plain text and consider using encryption and hashing algorithms for added protection.
Question 4: What are the common challenges associated with authentication systems?
Authentication systems can face challenges such as phishing attacks, brute-force attacks, and social engineering. Implementing strong authentication practices and staying informed about the latest threats is crucial for mitigating these risks.
Question 5: How can I improve the user experience while maintaining strong authentication?
Leveraging emerging authentication technologies like biometrics and behavioral analysis can enhance user convenience while maintaining robust security. These technologies offer alternative authentication methods that are both secure and user-friendly.
Question 6: What are the best practices for implementing authentication in C# applications?
Adhering to best practices such as enforcing strong passwords, implementing MFA, using secure storage for sensitive data, and following session management best practices is essential for building secure and reliable authentication mechanisms in C# applications.
In summary, understanding these FAQs provides valuable insights into the diverse aspects of authentication in C#, empowering developers to implement robust and secure authentication mechanisms in their applications.
Read further to explore advanced topics and trends in authentication using C#.
Authentication in C#
This article delved into the realm of authentication in C#, exploring its significance, methods, forms, and best practices. Authentication plays a pivotal role in safeguarding applications and systems by verifying the identity of users or entities, ensuring data security and access control.
We examined various authentication methods provided by C#, including password-based, biometric, and digital certificate authentication. Understanding the different forms of authentication, such as user authentication, service authentication, and device authentication, is crucial for addressing diverse authentication scenarios. Additionally, we highlighted the importance of strong authentication practices, such as implementing multi-factor authentication and adhering to secure password policies, to enhance the overall security of applications and systems.
Furthermore, we discussed challenges faced by authentication systems, including phishing attacks, brute-force attacks, and social engineering, and emphasized the need for developers to stay informed about evolving threats and trends. Emerging authentication technologies like biometrics and behavioral analysis are gaining traction, offering alternative authentication methods that are both secure and user-friendly.
In conclusion, authentication in C# is a multifaceted concept that requires a comprehensive understanding of its methods, forms, and best practices. By leveraging the capabilities of C# and implementing robust authentication mechanisms, developers can build secure and reliable applications and systems, safeguarding sensitive data and maintaining the integrity of their systems.
Uncover The Conversion: 80 Grams To Cups Of Sugar Made Easy
Ultimate Guide: **Tabulating The Distinctions Between Free And Economic Goods**
How Long Will ABS Glue Last?: Find Out Here
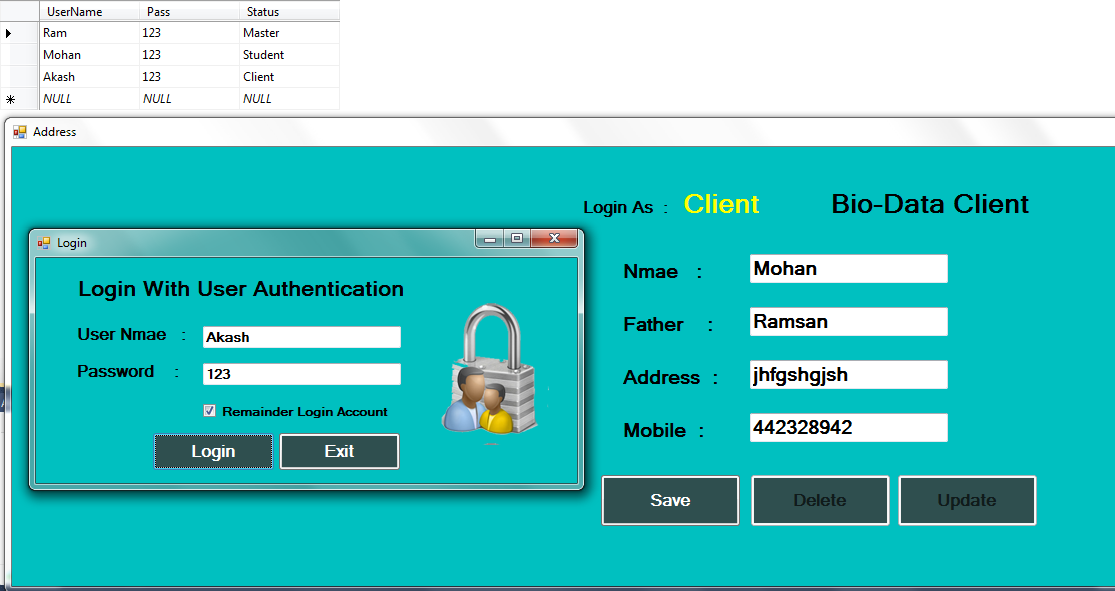
![[Solved] C SSL Basic Access Authentication 9to5Answer](https://i2.wp.com/sgp1.digitaloceanspaces.com/ffh-space-01/9to5answer/uploads/post/avatar/698516/template_c-ssl-basic-access-authentication20220613-1532174-1whcp1r.jpg)